react.animate alternatives and similar libraries
Based on the "UI Animation" category.
Alternatively, view react.animate alternatives based on common mentions on social networks and blogs.
-
react-flip-move
Effortless animation between DOM changes (eg. list reordering) using the FLIP technique. -
react-parallax-tilt
👀 Easily apply tilt hover effect on React components - lightweight/zero dependencies (3kB) -
React Native Circle Menu
:octocat: ⭕️ CircleMenu is a simple, elegant UI menu with a circular layout and material design animations. Reactnative library made by @Ramotion -
react-web-animation
React components for the Web Animations API - http://react-web-animation.surge.sh -
react-transitive-number
React component to apply transition effect to numeric strings, a la old Groupon timers -
react-tween
DISCONTINUED. DEPRECATED - Recommend https://github.com/tannerlinsley/react-move instead! -
anim-react
simple js react animation, animation hook, web Animation interface, onclick animation, onview,onsight animation, without css animation, no transition animation, js animation class usage.
SurveyJS - Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App
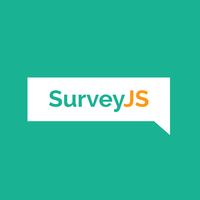
Do you think we are missing an alternative of react.animate or a related project?
README
React.Animate
A simple state animation mixin for React.js
Philosophy
React.Animate is a different approach to animate based on state rather than direct DOM mutation using $.animate or similar.
While it's great that you can use refs to get DOM nodes after render, the biggest benefit to using react is that there is always a direct, observable, and testable relationship between component props, state, and the rendered output.
Mutating the dom directly is an antipattern.
What we really want to animate is not the DOM, it's component state.
If you think about animation as a transition from one state value from another, you can just interpolate state over an interval, and your component can rerender precisely in response to the current component state at every step.
At it's most simple, React.Animate allows you to transition between one state and another over a set interval. The implementation supports the same syntax as $.animate.
you can pass either
this.animate(properties [, duration ] [, easing ] [, complete ] );
or
this.animate(key, value [, duration ] [, easing ] [, complete ] );
Example
React.Animate can be included in any React class by adding it to the mixins array
By animating state instead of the DOM directly, we can define logic that acts during certain parts of our animations.
var component = React.createClass({
mixins: [React.Animate],
getInitialState: function() {
return {
width: 100
};
},
render: function() {
var heightBounds = [50, 100];
return React.DOM.div({
style: {
width: this.state.width,
height: Math.min(heightBounds[1], Math.max(heightBounds[0], this.state.width / 2))
},
onClick: this.randomSize
});
},
randomSize: function() {
this.animate({
width: _.random(20, 300)
}, 500, function() {
console.log("random size reached!");
});
}
});
view in jsfiddle
Installation
React.Animate can be installed with bower using
bower install react.animate --save
React.Animate can be installed with npm using
npm install react.animate --save
Both will automatically pull the required React and Underscore dependencies.
to use React.Animate, include it in your page or build process after React and Underscore